vue 中图片可能只是用于展示,可能是裁剪,可能是点击图片进行跳转
图片加载失败
当获取的图片正常加载时对页面是没有影响的,但当图片加载失败时,可能会对布局造成影响或者是一个加载失败的图标(难看),所以要处理在图片加载问题
首先,要有个默认图片,图片url不存在时就显示这张,当图片存在但url无效时也显示这张:
1 2 3 4 5 6 7 8 9 10
| albumImgUnloadSrc: require('../../../assets/unload4.png'),
<img v-if="albumIcon" :src="albumIcon" @error="albumImgError"> <img v-else :src="albumImgUnloadSrc" @error="albumImgError">
albumImgError() { this.albumIcon = this.albumImgUnloadSrc; },
|
图片拖动及裁剪
有时会用图片拖动及裁剪,比如上传图片后获取其中一部分
现在假设上传的是一个1920x650的图片:
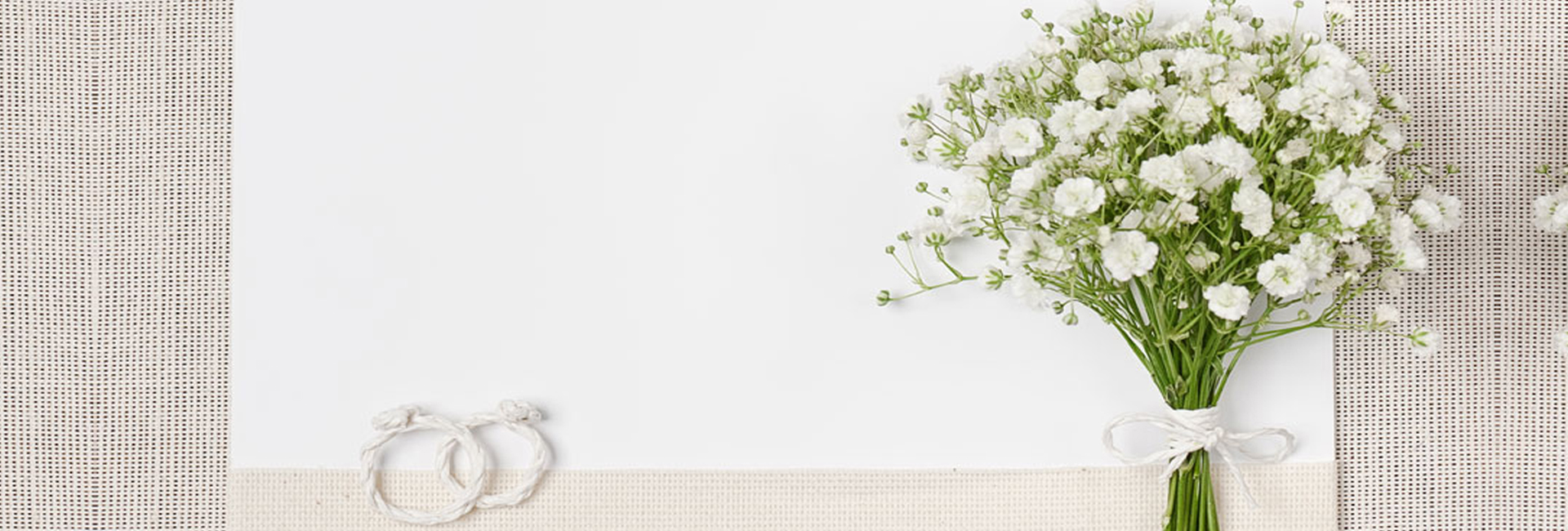
但现在要把它放在一个 325px 325px的div中,且拖动图片要展示相应的部分:
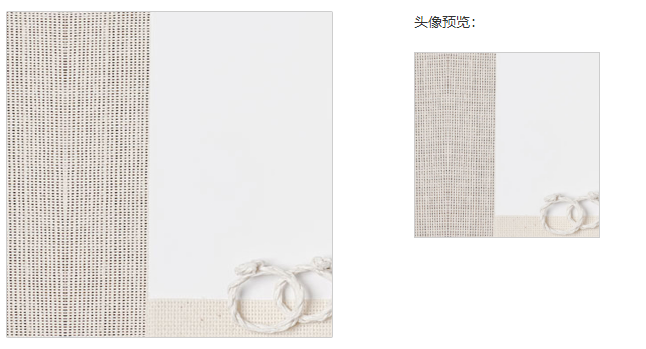
图片拖动的方法如下, x轴和y轴分开判断:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47
| <!-- 上传头像提示框 --> <div class="perIconChange-upIconK"> <canvas ref="cutCanvas" class="perIconChange-upCanvas" :style="cutIconStyle"</canvas> <img :src="pointIconSrc" alt="" :style="cutIconStyle" @mousedown.stop="iconDragMouse" draggable="false" ref="newCutImgEle"> <span class="perIconChange-upIconKT">选择一张本地的图片进行上传</span> </div>
//头像拖动 iconDragMouse(e) { let translate = parseInt(this.cutIconStyle.transform.slice(11)); let minuse = 0; if (this.iconDragBool === "x") { let originX = e.pageX; this.dragDistance = -Math.floor((325 / this.newIconHeight) * this.newIconWidth) + 325; document.onmousemove = e => { minuse = e.pageX - originX; translate = translate + parseInt(minuse); if (translate < 1 && translate > this.dragDistance) { this.cutIconStyle.transform = "translateX(" + translate + "px)"; } }; document.onmouseup = e => { console.log("up"); this.iconCutEvent(); document.onmousemove = null; document.onmouseup = null; }; } else if (this.iconDragBool === "y") { let originY = e.pageY; this.dragDistance = -Math.floor((325 / this.newIconWidth) * this.newIconHeight) + 325; document.onmousemove = e => { minuse = e.pageY - originY; translate = translate + parseInt(minuse); if (translate < 1 && translate > this.dragDistance) { this.cutIconStyle.transform = "translateY(" + translate + "px)"; } }; document.onmouseup = e => { this.iconCutEvent(); document.onmousemove = null; document.onmouseup = null; }; } },
|
图片裁剪,这个功能要和h5的canvas配合实现:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52
| iconCutEvent() { if (this.iconDragBool === "x") { this.canvasStyle = { x: Math.floor(this.newIconHeight / 325 * Math.abs(-parseInt(this.cutIconStyle.transform.slice(11)))), y: 0, w: this.newIconHeight, h: this.newIconHeight }; } else if (this.iconDragBool === "y") { this.canvasStyle = { x: 0, y: Math.floor(this.newIconWidth / 325 * Math.abs(-parseInt(this.cutIconStyle.transform.slice(11)))), w: this.newIconWidth, h: this.newIconWidth }; } else if (this.iconDragBool === '') { this.canvasStyle = { x: 0, y: 0, w: this.newIconWidth, h: this.newIconWidth }; } let canvas = document.createElement("canvas"); let ctx = canvas.getContext("2d"); this.$nextTick(() => { let imgData = this.$refs.cutCanvas .getContext("2d") .getImageData( this.canvasStyle.x * 2, this.canvasStyle.y * 2, this.canvasStyle.w * 2, this.canvasStyle.h * 2 ); canvas.width = this.canvasStyle.w * 2; canvas.height = this.canvasStyle.h * 2; ctx.putImageData( imgData, 0, 0, 0, 0, this.canvasStyle.w * 2, this.canvasStyle.h * 2 ); this.perHeadIconSrc = canvas.toDataURL("image/png"); }); },
|
图片的处理暂时到这里,以后碰到其他的再继续总结